Sanjivani intern Chaitanya Lokhande worked on this project. He completed his internship then this project was handover to me.
http://vadic.vigyanashram.blog/2022/01/03/automatic-gate-control-system-version-2/
Problem –
Chaitanya used a weighing balance mechanism for gate opening & closing. He used the delay function in Arduino Uno code for water fill-in to the attached gate and water fill-out to the reservoir. problem is that evaporation losses of water occur in summer that’s why the delay function does not work properly, so I decided to get feedback from a system.
Working –
I have used two float switches. one to check the open gate water level & another one to check the closed gate water level.
when the motor start to fill the water to the attached gate reservoir. if the water touches the float switch he sends a signal 1 to the ESP8266 & stopped a motor. Then the gate is opened.
when the motor start to fill out water to the gate reservoir. Then if the water below the float switch he sends a signal 0 to the Arduino & stopped the motor. Then the gate is closed.
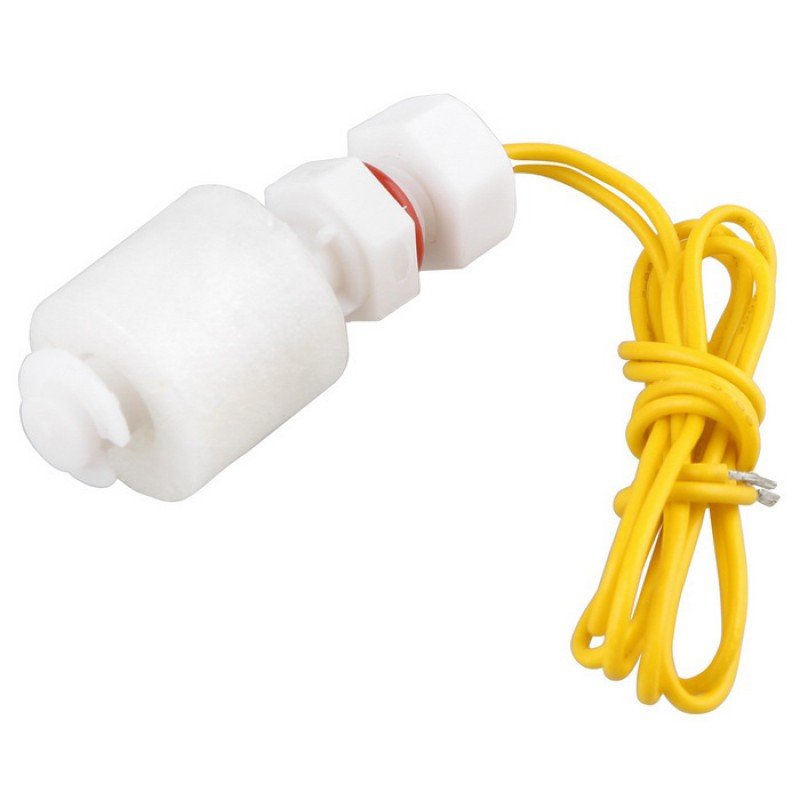
Pinout –
Sr.no | Components used | ESP8266 module |
1 | Float switch 1(open gate) | D4 |
2 | Float switch 2 (closed gate) | D6 |
3 | Relay 1 (pump in) | D7 |
4 | Relay 2(pump out) | D5 |
5 | Wi-Fi indication LED | RX |
6 | GND (common of all components) | GND |
7 | VCC (common of all components) | VCC |
Code –
#include <ESP8266WiFi.h>
#include "Adafruit_MQTT.h"
#include "Adafruit_MQTT_Client.h"
#define WLAN_SSID "VAIBT"//Vigyan New Office//Vigyan office 2//Workshop//VAIBT
#define WLAN_PASS "ibt412403" //rahul123456//vigyan@ashram30//VIGYAN0040//ibt412403
#define AIO_SERVER "io.adafruit.com"
#define AIO_SERVERPORT 1883
#define AIO_USERNAME "VAGateControl"
#define AIO_KEY "aio_ZCrs674uVZywEwF2q3JnUL9LINEe"
// Assign output variables to GPIO pins
const int water_pump_in = 13; //pin D7
const int water_pump_out = 14; // pin D5
//wifi indication
//const int wifi_indication = 16; // pin D0
const int wifi_indication = 3; // pin RX
// float sensor1
int FloatSensor1 = 2; //D4 pin of NodeMCU
// float sensor2
int FloatSensor2 = 12; //D6 pin of NodeMCU
//general declarations
int gate_state = 0;
int timer = 0;
WiFiClient client; // Create an ESP8266 WiFiClient class to connect to the MQTT server.
Adafruit_MQTT_Client mqtt(&client, AIO_SERVER, AIO_SERVERPORT, AIO_USERNAME, AIO_KEY);
// Setup the MQTT client class by passing in the WiFi client and MQTT server and login details.
Adafruit_MQTT_Subscribe GATE_CONTROL = Adafruit_MQTT_Subscribe(&mqtt, AIO_USERNAME"/feeds/GATE_CONTROL");
//Function declration
void MQTT_connect();
void open_gate ();
void closed_gate ();
void pump_in();
void setup() {
Serial.begin(115200);
delay(10);
//motor define
pinMode(water_pump_in, OUTPUT);
pinMode(water_pump_out, OUTPUT);
//wifi indication
pinMode(wifi_indication, OUTPUT);
// motor initially low
digitalWrite(water_pump_in, HIGH);
digitalWrite(water_pump_out, HIGH);
//float define
pinMode(FloatSensor1, INPUT); //Arduino Internal Resistor 10K
pinMode(FloatSensor2, INPUT_PULLUP); //Arduino Internal Resistor 10K
// wifi connected or not
Serial.println(); Serial.println();
Serial.print("Connecting to ");
Serial.println(WLAN_SSID);
WiFi.begin(WLAN_SSID, WLAN_PASS);
while (WiFi.status() != WL_CONNECTED)
{
delay(500);
Serial.print(".");
digitalWrite (wifi_indication, LOW);
}
Serial.println("WiFi connected");
digitalWrite (wifi_indication, HIGH);
Serial.println("IP address: "); Serial.println(WiFi.localIP());
mqtt.subscribe(&GATE_CONTROL);
}
uint32_t x = 0;
void loop()
{
MQTT_connect();
Adafruit_MQTT_Subscribe *subscription;
while ((subscription = mqtt.readSubscription(5000))) {
if (subscription == &GATE_CONTROL) {
Serial.print(F("Got: "));
Serial.println((char *)GATE_CONTROL.lastread);
// Open gate
if (!strcmp((char*) GATE_CONTROL.lastread, "ON") && gate_state == 0)
{
open_gate();
}
//Close gate
else if (!strcmp((char*) GATE_CONTROL.lastread, "OFF") && gate_state == 1)
{
closed_gate();
delay(66000);//1.1 min for gate closing
pump_in();
}
}
}
}
delay(1000);
void MQTT_connect() {
int8_t ret;
// Stop if already connected.
if (mqtt.connected()) {
return;
}
Serial.print("Connecting to MQTT... ");
uint8_t retries = 3;
int cnt = 5;
while ((ret = mqtt.connect()) != 0) { // connect will return 0 for connected
Serial.println(mqtt.connectErrorString(ret));
Serial.println("Retrying MQTT connection in 5 seconds...");
mqtt.disconnect();
delay(5000); // wait 5 seconds
/* retries--;
// reset command
if (retries == 0) {
while (1);
}*/
// basically die and wait for WDT to reset me
// ESP.restart();
//Serial.println("Reset..");
//delay(1000);
}
Serial.println("MQTT Connected!");
}
void open_gate()
{
Serial.println(digitalRead (FloatSensor1));
while (digitalRead(FloatSensor1) == 0)
{
digitalWrite(water_pump_in, LOW);
Serial.println(digitalRead (FloatSensor1));
}
while (digitalRead(FloatSensor1) == 1)
{
digitalWrite(water_pump_in, HIGH);
Serial.println(digitalRead (FloatSensor1));
break;
}
delay(100);
gate_state = 1;
Serial.println("Gate Open");
}
void closed_gate ()
{
Serial.println(digitalRead (FloatSensor2));
while (digitalRead(FloatSensor2) == 1)
{
digitalWrite(water_pump_out, LOW);
Serial.println(digitalRead (FloatSensor2));
}
while (digitalRead(FloatSensor2) == 0)
{
digitalWrite(water_pump_out, HIGH);
Serial.println(digitalRead (FloatSensor2));
break;
}
delay (100);
gate_state = 0;
}
void pump_in()
{
// water pump in ON
if((digitalRead(FloatSensor1) == 0)&& (digitalRead(FloatSensor2) == 0))
{
for (timer = 0; timer < 90; timer++) //
{
digitalWrite(water_pump_in, LOW);
Serial.println( "Pump in ON");
delay(1000);//1 seconds
}
}
digitalWrite(water_pump_in, HIGH);
Serial.println( "Pump in OFF");
timer = 0;
delay(10);
Serial.println("Gate Close");
}
Observations –
A float switch was placed in that system is working properly without problem.
IFTTT is an MQTT broker used for giving voice commands to Google Assistance.
But after some months IFTTT policies were changed. I read these policies & I found about IFTTT access is going on after paying some amount, that’s why I am finding another option for that. After some time discussing with Suhas sir , when we got one solution which is to make a graphic user interface (GUI) for Vigyan ashram gate & this task was given to an intern Basile Pasqual which was come from France.