Problem Statement :
Design and development of a device/system for odour detection in a toilet for the safe and hygienic surrounding.
Ideation :
So I come up with the concept of being able to detect the smell of NH3 in order to install an exhaust fan in the toilet and also provide certain indicators, such as how much odour arrives, and then I set the limit to the indication in accordance with that. If the sensor detects a HIGH level of NH3 (in ppm), we should clean our toilet based on that indication.
So basically, only the urea(Carbamide) is present in fresh human urine, and the odour is caused by contamination with bacteria that break down urea into ammonia. So I decide to sense the NH3 gas using the MQ-137 NH3 sensor.
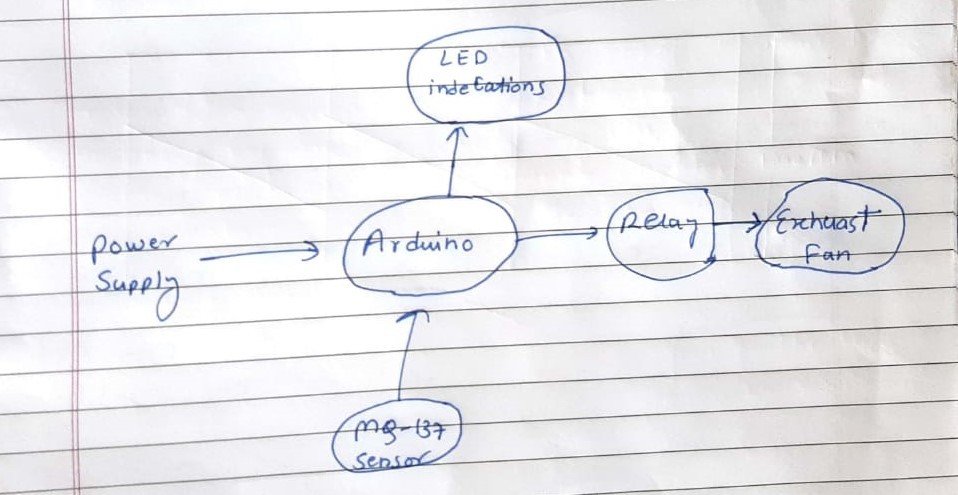
Process flow of project
Component I need :
Approach to Measure PPM from MQ-137 Gas Sensors:
We just need to connect the RL (load register) between input and GND. The best value for RL will be 47K as suggested by datasheet hence we are going to use the same. Now that we know the value of RL lets proceed on how to actually measure ppm from these sensors. Like all sensors the place to start is its datasheet. The MQ-137 Datasheet is given here but make sure you find the correct datasheet for your sensor. Inside the datasheet we are need only one graph which will be plotted against (Rs/Ro) Vs ppm this is the one that we need for our calculations. So grab it and keep it someplace handy. The one for my sensor is shown below.
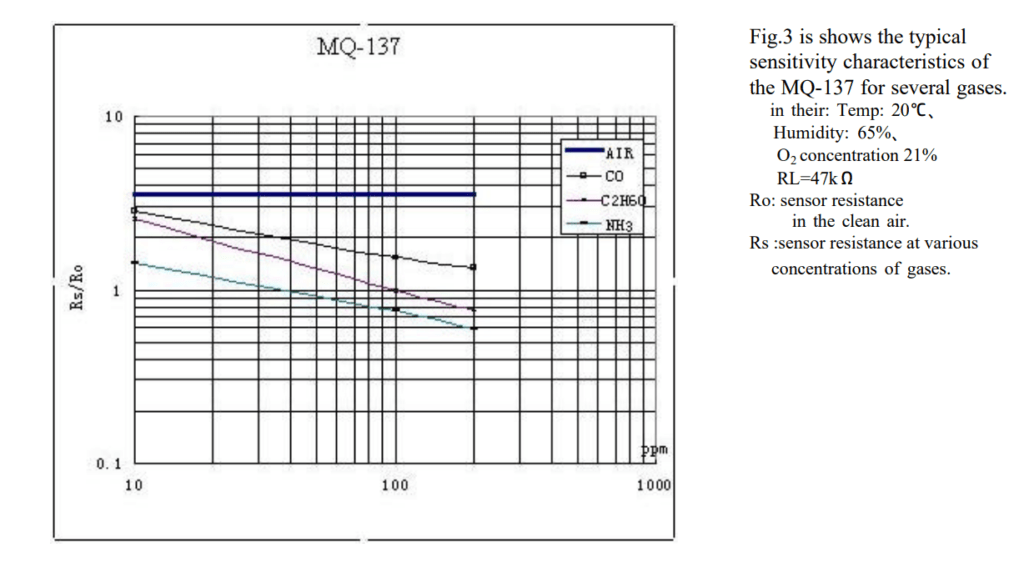
Sensitivity Characteristics of the MQ-137
So from the datasheet, we can see that this sensor can also show some sensitivity to CO as well as C2H6O. But I’m only interested in sensing NH3. However you can use the same method to calculate ppm for any sensor you like. This graph is the only source for us to find the value of ppm and if we could somehow calculate the ration of Rs/Ro (X-axis) we can use this graph to find the value of ppm (Y-axis). To find the value of Rs/Ro we need to find the value of Rs and the value of Ro. Where Rs is the Sensor resistance at gas concentration and Ro is the sensor resistance in clean air. So let’s see …
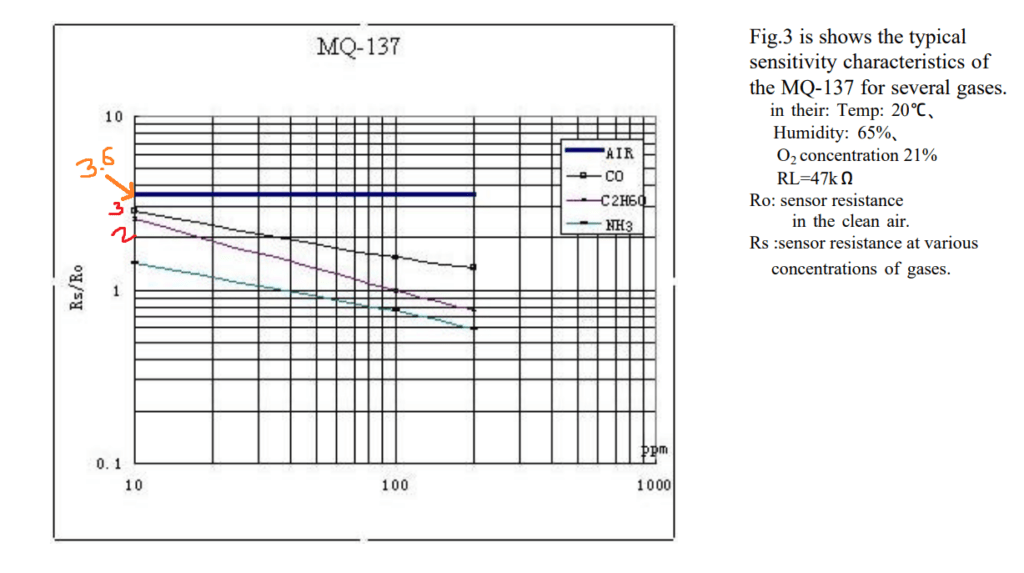
Value of Rs/Ro at Fresh air according to datasheet
From the datasheet of the MQ-137 we also get to have a formula for calculating the value of Rs. The formula is shown below. If anyone is interested to know how the formula is derived then you can read the blog of jaycon systems. This blog help me a lot for doing calculations.
Sensor resistor i.e. Rs = (VC/VRL -1) x RL
In this formula the value of Vc is our supply voltage (+5V) and the value of RL is the one that we calculated already (47K for my sensor). If we write a small Arduino program we could also find the value of VRL and finally calculate the value of Rs. I have given an Arduino Program below which reads the analog voltage (VRL) of the sensor and calculates the value of Rs using this formula and finally displays it in the serial monitor. I have explained the program below in comment section.
Program to measured Ro :
/*
* Program to measure the value of R0 for a know RL at fresh air condition
* Program by: Somnath Bhusari
*/
//This program works best at a fresh air room with temperature Temp: 20℃, Humidity: 65%, O2 concentration 21% and when the value of RL is 47K
#define RL 47 //The value of resistor RL is 47K
void setup() //Runs only once
{
Serial.begin(9600); //Initialize serial COM for displaying the value
}
void loop() {
float analog_value;
float VRL;
float Rs;
float Ro;
for(int test_cycle = 1 ; test_cycle <= 500 ; test_cycle++) //Read the analog output of the sensor for 200 times
{
analog_value = analog_value + analogRead(A0); //add the values for 200
}
analog_value = analog_value/500.0; //Take average
VRL = analog_value*(5.0/1023.0); //Convert analog value to voltage
//RS = ((Vc/VRL)-1)*RL is the formulae we obtained from datasheet
Rs = ((5.0/VRL)-1) * RL;
//RS/RO is 3.6 as we obtained from graph of datasheet
Ro = Rs/3.6;
Serial.print("Ro at fresh air = ");
Serial.println(Ro); //Display calculated Ro
delay(1000); //delay of 1sec
}
Note: The value of Ro will be varying, allow the sensor to pre-heat at least for 10 hours and then use the value of Ro.
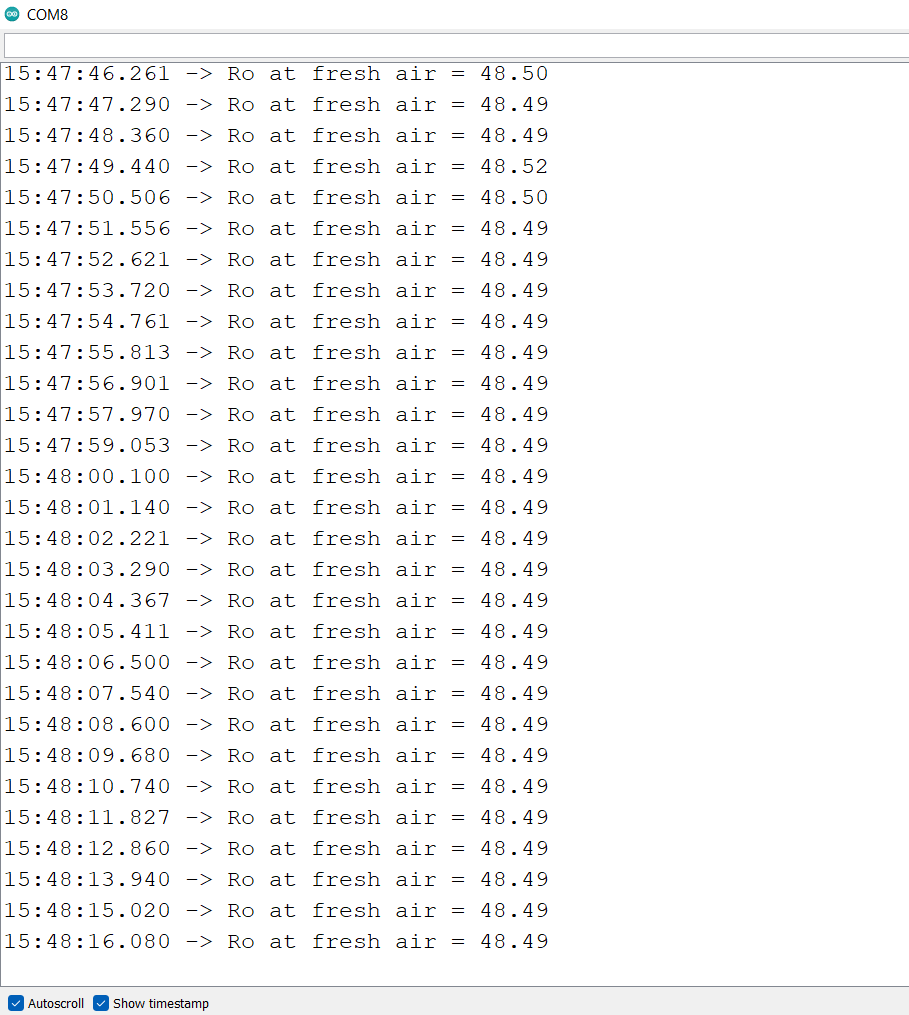
Ro Value at fresh air
The value should be depend on environment like Temp., Humidity, O2 Concentration, etc.
Measuring the value of Rs :
Now that we know the value of Ro we can easily calculate the value of Rs using the above two formulae. Note that the value of Rs that was calculated previously is for fresh air condition and it will not be the same when ammonia is present in the air. Calculating the value of Rs is not a big issue which we can directly take care in the final program.
Calculating theoretical value of Rs/Ro :
Now that we know how to measure the value of Rs and Ro we would be able to find its ratio (Rs/Ro). Then we can use the graph (shown below) to relate to the corresponding value of PPM.
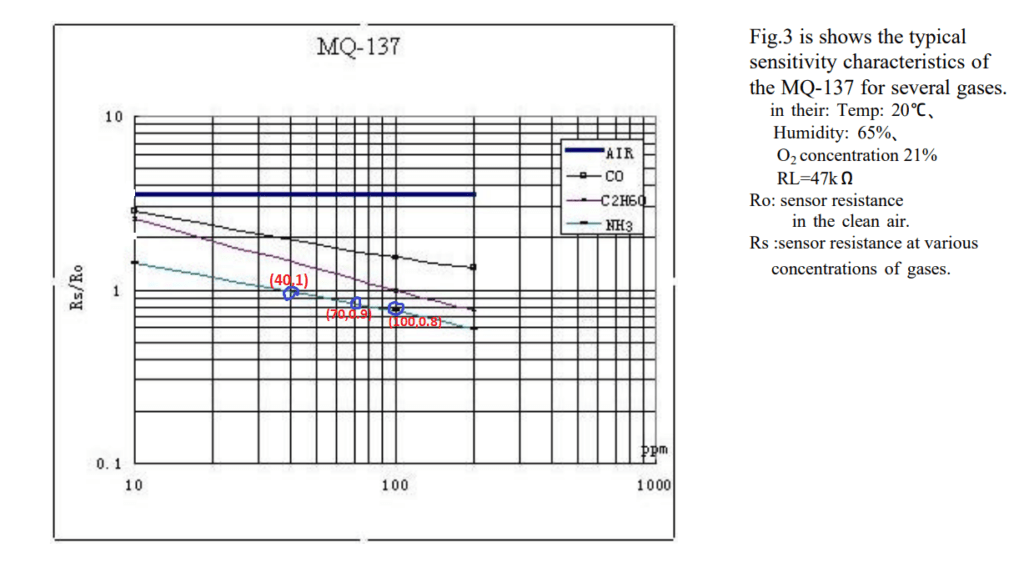
Calculating the value of ratio (Rs/Ro)
So the NH3 line showed in cyan colour. So the relation between Rs/Ro and PPM is actually logarithmic which can be represented by the below equation.
log(y) = m*log(x) + b
where,
y = ratio (Rs/Ro)
x = PPM
m = slope of the line
b = intersection point
To find the values of m and b we have to consider two points (x1,y1) and (x2,y2) on our gas line. Here we are working with ammonia so the two points I have considered is (40,1) and (100,0.8) as shown in the picture above (marked as red) with red marking.
m = [log(y2) - log(y1)] / [log(x2) - log(x1)]
m = log(0.8/1) / log(100/40)
m = -0.243
Similarly for (b) let’s get the midpoint value (x,y) from the graph which is (70,0.9) as shown in picture above (marked in blue).
b = log(y) - m*log(x)
b = log(0.9) - (-0.243)*log(70)
b = 0.402
That’s it now that we have calculated the value of m and b we can equate the value of (Rs/Ro) to PPM using the below formula.
PPM = 10 ^ {[log(ratio) - b] / m}
Program to calculate the PPM using MQ-137 Sensor :
Before proceeding with the program we need to feed in the values of Load resistance (RL), Slope(m), Intercept(b) and the value of Resistance in fresh air (Ro). The procedure to obtain all these values have already be explained above.
#define RL 47 //The value of resistor RL is 47K
#define m -0.263 //Enter calculated Slope
#define b 0.402 //Enter calculated intercept
#define Ro 48 //Enter found Ro value
Then read the Voltage drop across the sensor (VRL) and convert it to Voltage (0V to 5V) since the analog read will only return values from 0 to 1024.
VRL = analogRead(MQ_sensor)*(5.0/1023.0); //Measure the voltage drop and convert to 0-5V
Now, that the value of VRL is calculated you can use the formula discussed above to calculate the value of Rs and the also the ratio (Rs/Ro).
ratio = Rs/Ro; // find ratio Rs/Ro
Finally, we can calculate the PPM with our logarithmic formula and display it on our serial monitor as shown below.
double ppm = pow(10, ((log10(ratio)-b)/m)); //use formula to calculate ppm
Serial.print(ppm); //Display ppm
Circuit Diagram :
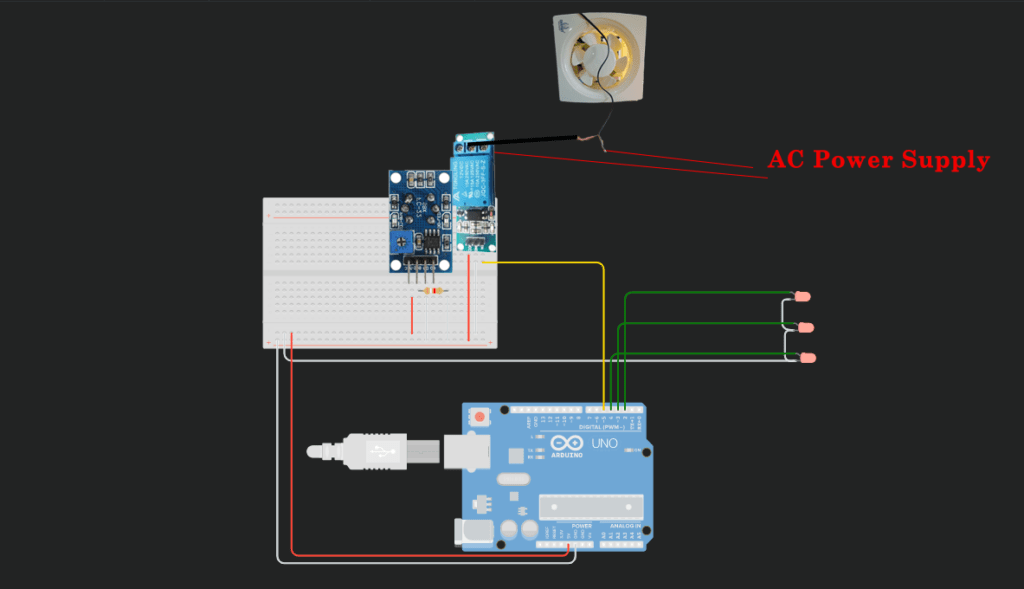
Circuit Diagram
Final Code:
So I have written the logic of code in the comment, You can read it.
#define RL 47 //The value of resistor RL is 47K
#define m -0.263 //Enter calculated Slope
#define b 0.402 //Enter calculated intercept
#define Ro 48 //Enter found Ro value
#define MQ_sensor A0 //Sensor is connected to A4
#define RED 2 //Enter the red led pin
#define GREEN 3 //Enter the green led pin
#define BLUE 4 //Enter the blue led pin
#define relay_pin 5 //Enter relay pin
#include <LiquidCrystal.h>
const int rs = 8, en = 9, d4 = 10, d5 = 11, d6 = 12, d7 = 13; //Pins to which LCD is connected
LiquidCrystal lcd(rs, en, d4, d5, d6, d7);
void setup() {
Serial.begin(9600);
lcd.begin(16, 2); //We are using a 16*2 LCD display
lcd.print("NH3 in PPM"); //Display a intro message
Serial.print("NH3 in PPM");
lcd.setCursor(0, 1); // set the cursor to column 0, line 1
lcd.print("Somnath Bhusari"); //Display a intro message
Serial.print("Somnath Bhusari");
delay(1000); //Wait for display to show info
lcd.clear(); //Then clean it
pinMode(RED, OUTPUT);
pinMode(GREEN, OUTPUT);
pinMode(BLUE, OUTPUT);
}
void loop() {
float VRL; //Voltage drop across the MQ sensor
float Rs; //Sensor resistance at gas concentration
float ratio; //Define variable for ratio
VRL = analogRead(MQ_sensor)*(5.0/1023.0); //Measure the voltage drop and convert to 0-5V
Serial.print("MQ Sensor: ");
Serial.print(MQ_sensor);
Serial.print("VRL=");
Serial.print(VRL);
Rs = ((5.0*RL)/VRL)-RL; //Use formula to get Rs value
ratio = Rs/Ro; // find ratio Rs/Ro
Serial.print("RAtio=");
Serial.print(ratio);
float ppm = pow(5, ((log10(ratio)-b)/m)); //use formula to calculate ppm
float ppm1 = ppm * 10;
lcd.print("NH3 (ppm) = "); //Display a ammonia in ppm
Serial.print("NH3 (ppm) = ");
lcd.print(ppm1);
Serial.println(ppm1);
lcd.setCursor(0, 1); // set the cursor to column 0, line 1
lcd.print("NH3 is : ");
Serial.print("NH3 is : ");
//This is a main logic of the code:-
if(ppm1 <= 5.00){ //if the ppm of NH3 less than 5.0 then no problem
digitalWrite(3, HIGH);
lcd.print("LOW");
digitalWrite(4, LOW);
digitalWrite(2, LOW);
Serial.println("LOW");
digitalWrite(5, LOW);
}
else if(5.10 <= ppm1 && ppm1<= 10.00){ //if the ppm of NH3 is in between 5.1-10.0 then exhaust fan will start
digitalWrite(4, HIGH);
lcd.print("MEDIUM");
digitalWrite(3, LOW);
digitalWrite(2, LOW);
Serial.println("MEDIUM");
digitalWrite(5, HIGH);
}
else if(10.10 < ppm1){ //if the ppm of NH3 more than 10 then we need to clean the toilet
digitalWrite(2, HIGH);
lcd.print("HIGH");
digitalWrite(3, LOW);
digitalWrite(4, LOW);
Serial.println("HIGH");
digitalWrite(5, HIGH);
}
delay(500);
lcd.clear(); //Then clean lcd
}
Final Design file:
This is the casing for my project.
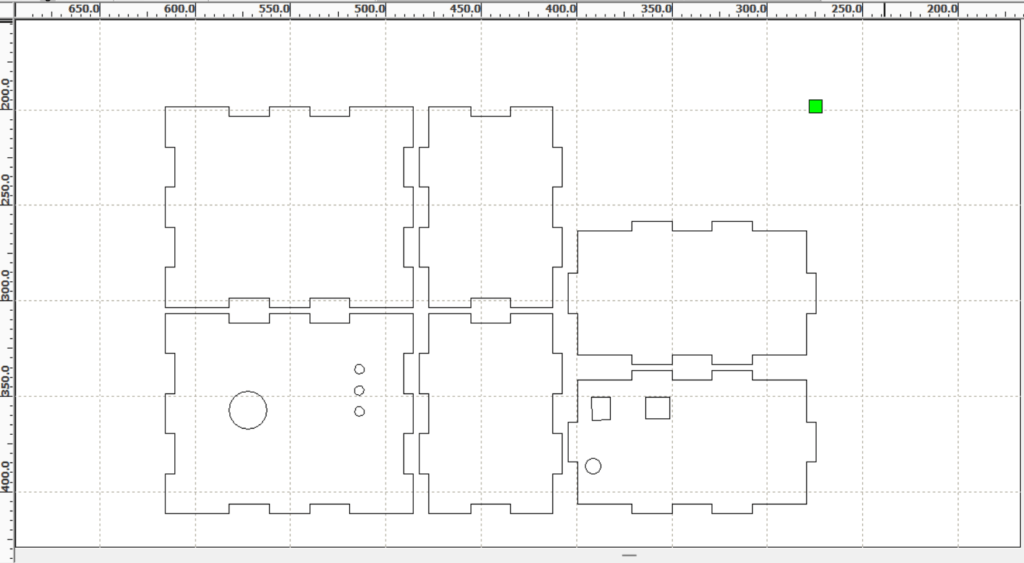
Final Casing
Click Here to download this files.
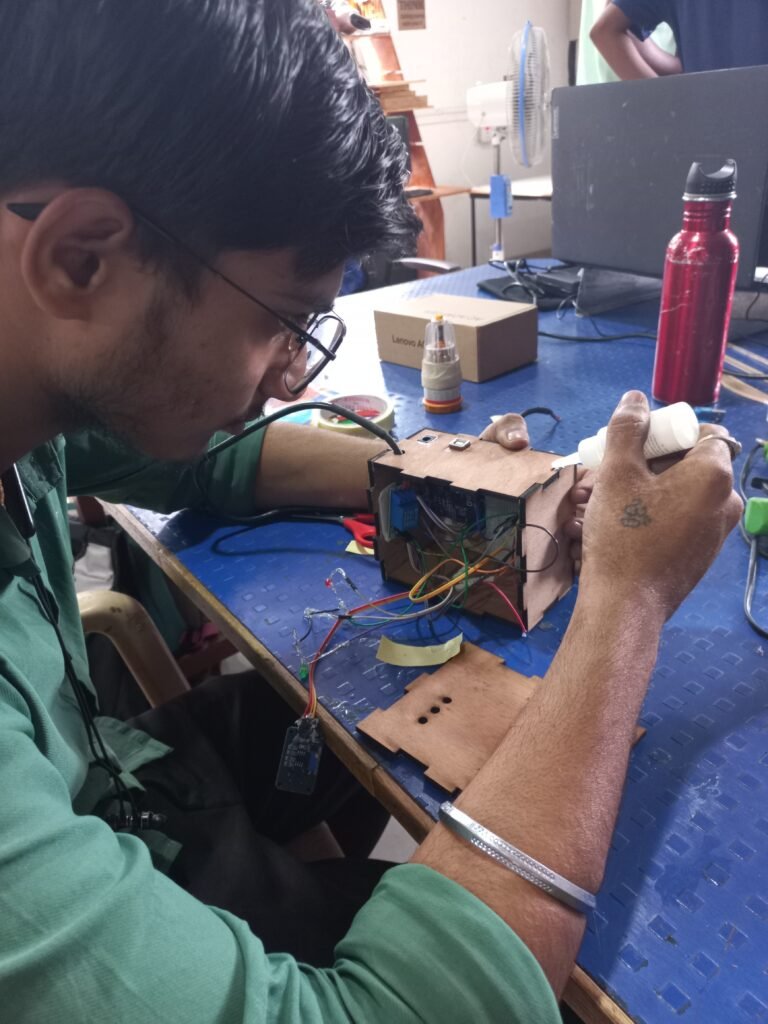
Giving Final Finishing
Final Photo:
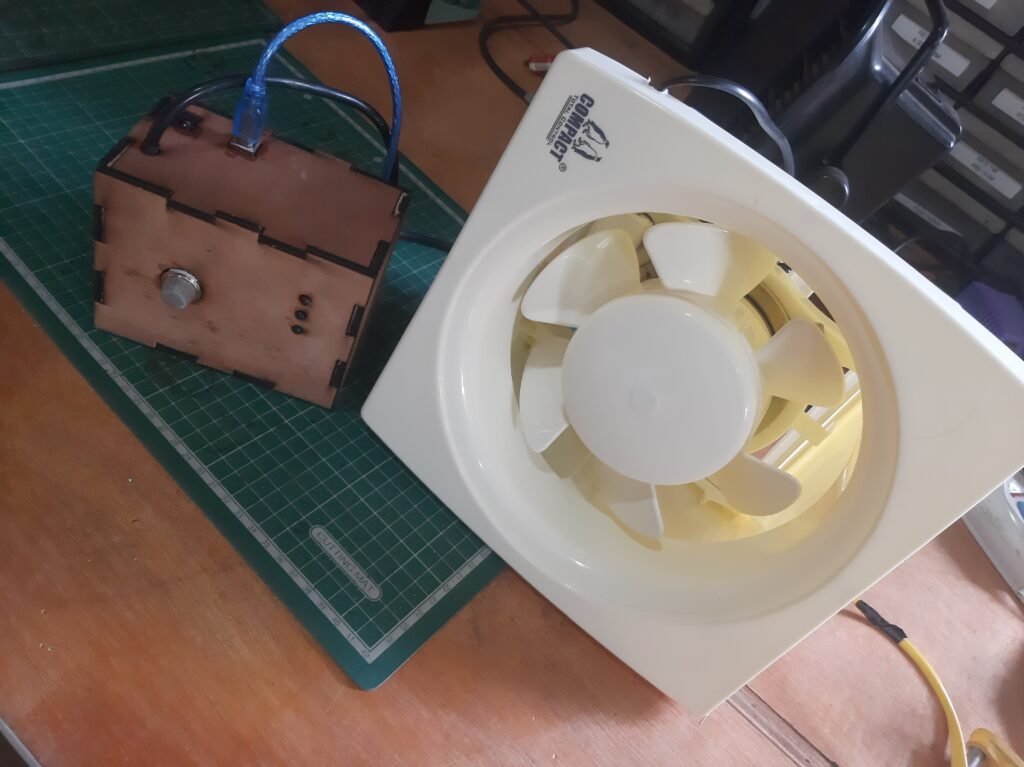
Final Photo