A timer bell is a device that is used to measure a specific duration of time and notify the user when that time has elapsed. This device can be used for a wide range of activities that require precise timing, such as cooking, exercise, or even for managing a daily routine. At the Vigyan Ashram, the timer bell is utilized to indicate the timing of various daily activities, such as lunch, prayer, meditation, and other routine tasks. The timer bell is an important device for managing time effectively and ensuring that routine tasks are carried out efficiently. By setting the timer for a specific duration, the user can focus on the task at hand without having to constantly check the time, and the bell will notify them once the set time has elapsed. This device is simple to use, effective, and can be found in a wide range of settings. The timer bell works by using an electric mechanism that consists of electromagnets and an iron rod that hits the bell repeatedly to generate a ringing sound. The device activates the electromagnets, which then pull the iron rod towards the bell. As the iron rod collides with the bell, it creates a distinct sound that alerts the user that the set duration of time has elapsed. Previously there have been a few DIC students who have worked on this problem and attempted to automate the timer bell using timers such as TS1W1 and microcontroller ESP8266 with RTC module or NTP servers. In spite of this these attempts the timer bell has stopped working.
Links to the previous blogs are given below:
- https://vadic.vigyanashram.blog/2020/06/06/electric-bell/
- https://vadic.vigyanashram.blog/2020/12/28/automatic-bell-system/
- https://vadic.vigyanashram.blog/2021/06/29/automatic-bell-2/
Solution
In order to solve the problem and get the bell working again I used an ESP32 microcontroller with NTP server that was obtained from WiFi. The current time is taken from the WIFI and then the ESP32 continues to count the time using it’s internal clock. The ESP32 activated a 5V relay that switched on and off the AC supply going to the electric bell at the required time. An indicator LED was used to show to status of the device. If the LED is on the device is connecting to the WiFi or the time is not obtained. In order to make the code readable and reusable a few provisions were made in the code.
//Include libraries
#include <WiFi.h>
#include "time.h"
int statled = 19;
//Define variables
int h;int m;int s;int t;
const int buzzer = 14;
//***********************++++++**To be changed and decided by the user********************************************//
const int ringing_timer = 3;//Time for whcih the bell will ring (in seconds)
int belltime[] = {645, 655, 900, 925, 1300, 1400, 1645, 1940,-1}; //Times at which the bell should ring (Format: hhmm) with -1 at the end to show end of the array
//Enter the wifi details
const char* ssid = "WIFI SSID"
const char* password = "PASSWORD";
//Data changed according to time zone
const char* ntpServer = "pool.ntp.org";
const long gmtOffset_sec = 19800;
const int daylightOffset_sec = 0;
void printLocalTime()
{
//store time info in a structure
struct tm timeinfo;
for(;!getLocalTime(&timeinfo);)
{
Serial.println("Failed to obtain time");
digitalWrite(statled, HIGH);
delay(2000);
return;
}
digitalWrite(statled, LOW);
//Store time in variables
h = timeinfo.tm_hour;
m = timeinfo.tm_min;
s = timeinfo.tm_sec;
t = (h*100)+(m);
}
void setup()
{
//initiate the buzzer output pins
pinMode(statled, OUTPUT);
pinMode(buzzer, OUTPUT);
digitalWrite(buzzer, LOW);
delay(50);
digitalWrite(buzzer, HIGH);
Serial.begin(115200);
//connect to WiFi
Serial.printf("Connecting to %s ", ssid);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
digitalWrite(statled, HIGH);
}
Serial.println(" CONNECTED");
digitalWrite(statled, LOW);
//init and get the time
configTime(gmtOffset_sec, daylightOffset_sec, ntpServer);
printLocalTime();
//disconnect WiFi as it's no longer needed
WiFi.disconnect(true);
WiFi.mode(WIFI_OFF);
}
void loop()
{
delay(500);
printLocalTime();
int c_time = 1;
for(int i = 0; c_time != -1; i++)
{
c_time = belltime[i];
Serial.println(t);
//check for the time and ring the bell
if(t == c_time && s >0 && s<ringing_timer)
{
Serial.print(t);Serial.println(s);
digitalWrite(statled, HIGH);
digitalWrite(buzzer, LOW);
delay(ringing_timer*1000);
digitalWrite(buzzer, HIGH);
digitalWrite(statled, LOW);
}
}
}
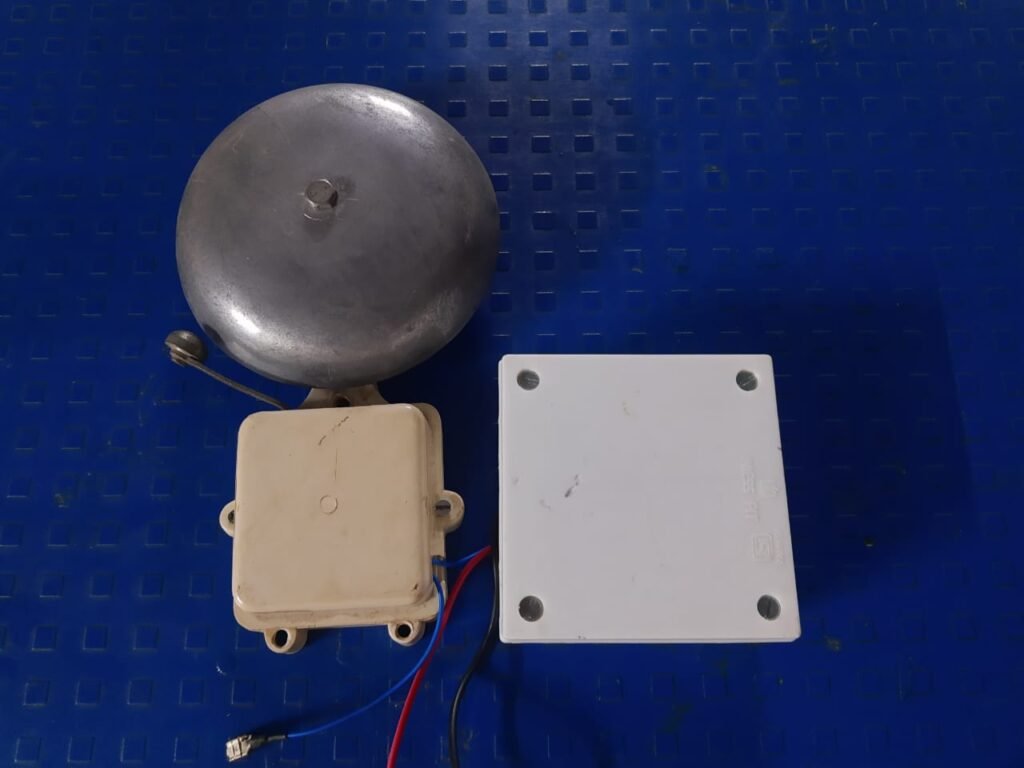
The Timer bell was installed and checked. It is working as expected.