Problem Statement:
To automate bell system in Vigyan Ashram which is done manually .
Proposed Solution:
This problem can be solved using two ways according to convenience of end user. Although the second approach is more cost effective .
1.Using ESP-8266(NodeMCU)
2. Using Timer Selec TS1W1
1. ESP8266:
For Automation of bell system we need to fetch time either from RTC(Real Time Clock) or Internet(Using NTP Server) . But RTC clock of ESP is not reliable as it gives delay after some time . So, we will use NTP Server(Network Time Protocol) . First we will connect esp to internet using network module . I have used Micropython for programming ESP and uPycraft IDE . Code is as follows:
import network
wlan = network.WLAN(network.STA_IF)
wlan.active(True)
wlan.connect(“SSid”,”Password”)
You can check if esp is connected to wifi using command “wlan.isconnected()” . It will return boolean value True or False . Once we are connected to wifi , we can fetch time using NTP module . We will fetch Time and save it into RTC
import ntptime
from ntptime as settime
rtc = RTC()
rtc.datetime()
settime()
a=rtc.datetime()
After fetching time , we will loop this code to fetch continuosly using while loop .
Also for giving signal to bell we will use relay of 5V. We have to define relay using relay variable . We will use GPIO 14 (D5) of ESP 8266 for relay .
relay = Pin(14 , Pin.OUT)
For ringing bell at specific time we will check time we have fetched and then give signal to relay to turn on and off in specific pattern.
if a[4] == 5 and a[5] == 30 and a[6]==0:
for i in range(10):
relay.value(0)
time.sleep(0.5)
relay.value(1)
time.sleep(0.5)
Above I have given code in different parts for understanding Here is all combined code:
import network
import time
import ntptime
from machine import RTC,Pin
from ntptime import settime
relay = Pin(14, Pin.OUT)
relay.value(1)
led=Pin(2,Pin.OUT)
led.value(1)
wlan = network.WLAN(network.STA_IF)
wlan.active(True)
wlan.connect(“Your SSID”,”Password”)
time.sleep(3)
rtc = RTC()
rtc.datetime()
settime()
a=rtc.datetime()
def bell_time():
a=rtc.datetime()
print(a[4],” “,a[5],” “,a[6])
if a[4] == 5 and a[5] == 30:
for i in range(10):
relay.value(0)
time.sleep(0.5)
relay.value(1)
time.sleep(1)
if a[4] == 6 and a[5] == 30:
for i in range(10):
relay.value(0)
time.sleep(0.5)
relay.value(1)
time.sleep(1)
if a[4] == 7 and a[5] == 10:
for i in range(10):
relay.value(0)
time.sleep(0.5)
relay.value(1)
time.sleep(1)
if a[4] == 1 and a[5] == 0:
for i in range(10):
relay.value(0)
time.sleep(0.5)
relay.value(1)
time.sleep(1)
if a[4] == 2 and a[5] == 0:
for i in range(10):
relay.value(0)
time.sleep(0.5)
relay.value(1)
time.sleep(1)
if a[4] == 8 and a[5] == 30:
for i in range(10):
relay.value(0)
time.sleep(0.5)
relay.value(1)
time.sleep(1)
if a[4] == 11 and a[5] == 30:
for i in range(10):
relay.value(0)
time.sleep(0.5)
relay.value(1)
time.sleep(1)
if a[4] == 12 and a[5] == 0:
for i in range(10):
relay.value(0)
time.sleep(0.5)
relay.value(1)
time.sleep(1)
if a[4] == 13 and a[5] == 25:
for i in range(10):
relay.value(0)
time.sleep(0.25)
relay.value(1)
time.sleep(1)
if a[4] == 14 and a[5] == 15:
for i in range(10):
relay.value(0)
time.sleep(0.5)
relay.value(1)
time.sleep(1)
while True:
if(wlan.isconnected()):
led.value(0)
else:
led.value(1)
wlan.connect(“SSID”,”password”)
bell_time()
time.sleep(0.1)
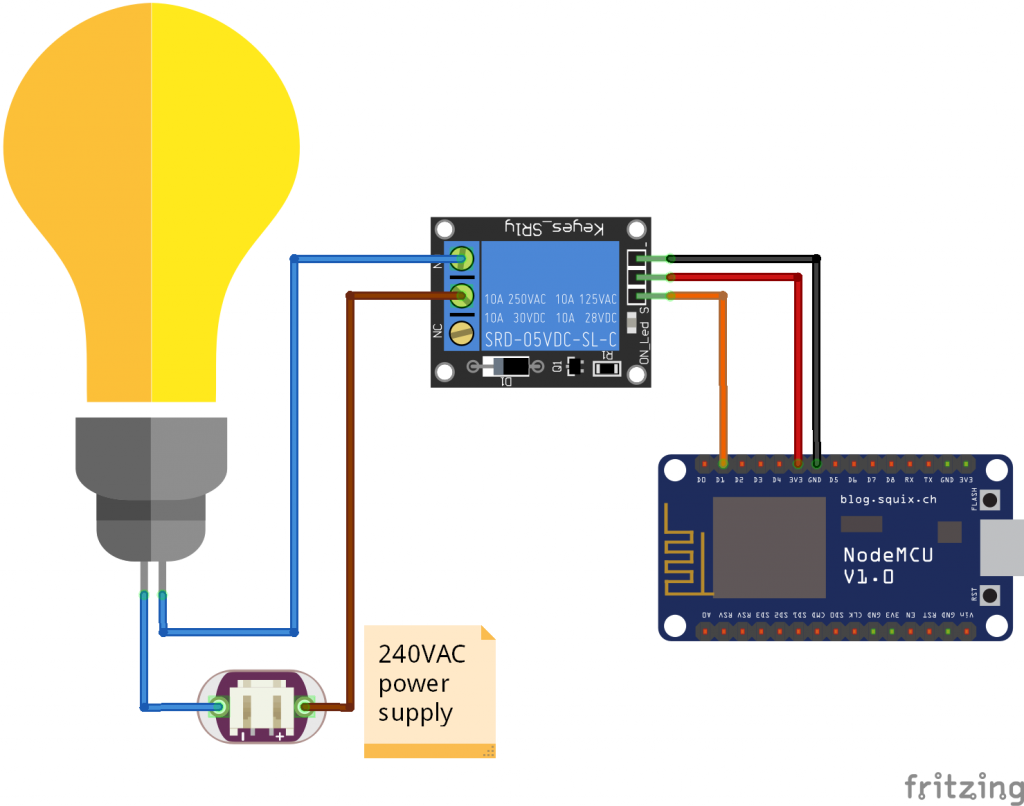
2. Timer (Selec TS1W1):
Using Timer for Automatic Bell System is more easy & reliable .We can give upto 50 alarms on this timer .
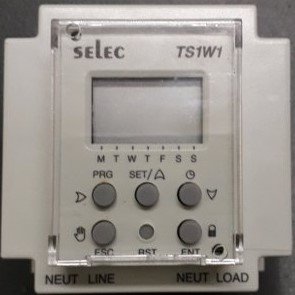
- First reset timer by pressing reset button and holding it for 5 sec.
- Set current day , date and time using third button in first row.
- Then for setting Alarm press program button (First button in first row)
- Select the function & day on which you want to set alarm.
- Enter the time on which bell or alarm should ring and also set time for which bell should ring.
- After setting alarm , you can set more alarms pressing enter after first alarm & so on .
- Connections of timer are similar to that of relay .
- Give AC supply to the neutral & line pins on timer & connect bell to neutral and load on other side .
Errors during Project:
Most of the errors were due to wrong connections and some manual errors in code. Also RTC clock of ESP does not work properly . For NTP time it cannot fetch time every second as complexity increases too much .
Another error I faced was interfacing relay . Use relay variable in code , another variables do not work .