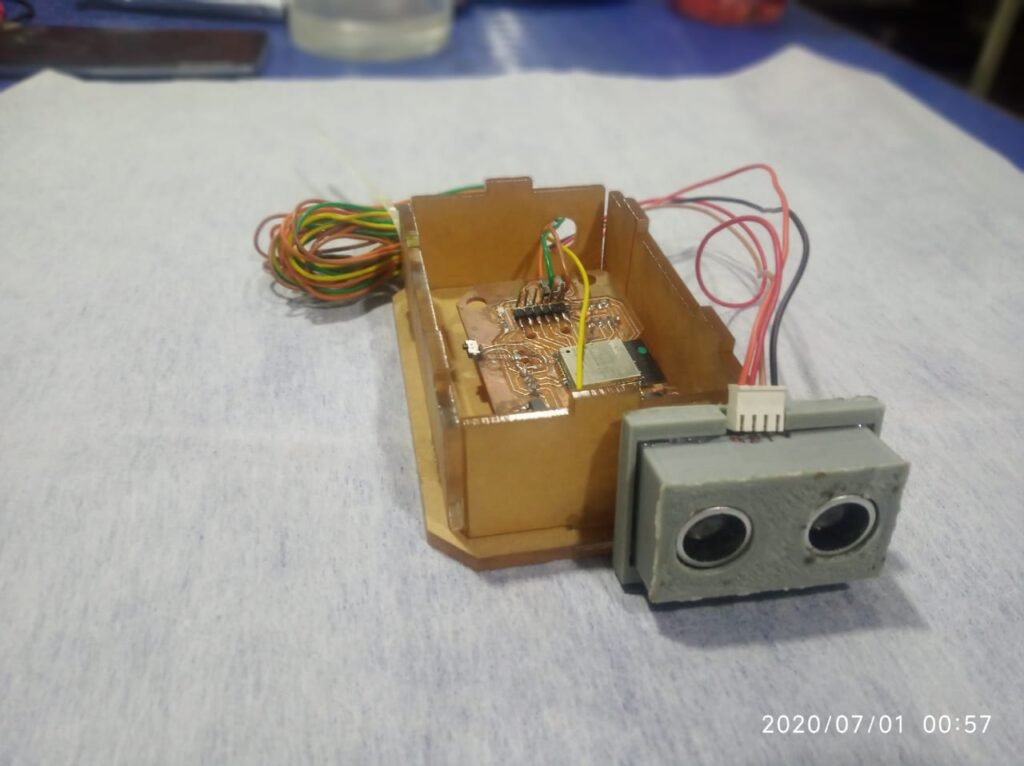
Problem Statment: In Vigyan Ashram,we have number of water tank to fulfill our daily water requirement ,but some times tank gets overfull ,hence lots of water wastage.My project is to develop a IOT system that can monitor the level of water and notify it on our mobile.
In Current scenario Snehal Gawali have devloped a basic system as her Fab academy project , and my task is to modify the loopholes and install the system at ladies hostel.
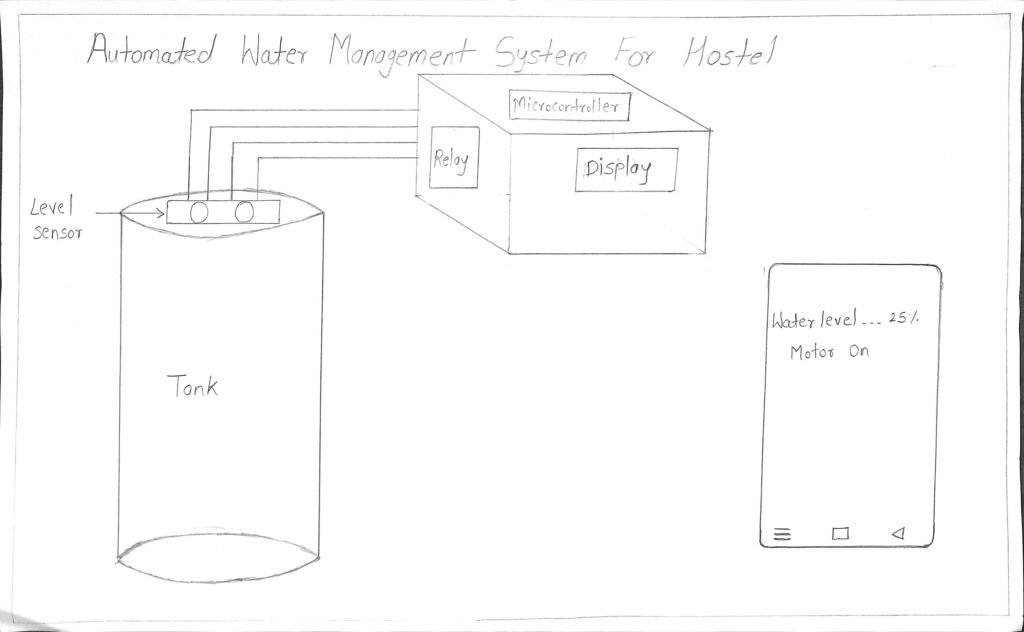
Components used:
- ESP -32 microcontroller
- Ultrasonic/Distance sensor
- 5v ,1 A Adapter
Why ESP-32 microcontroller?
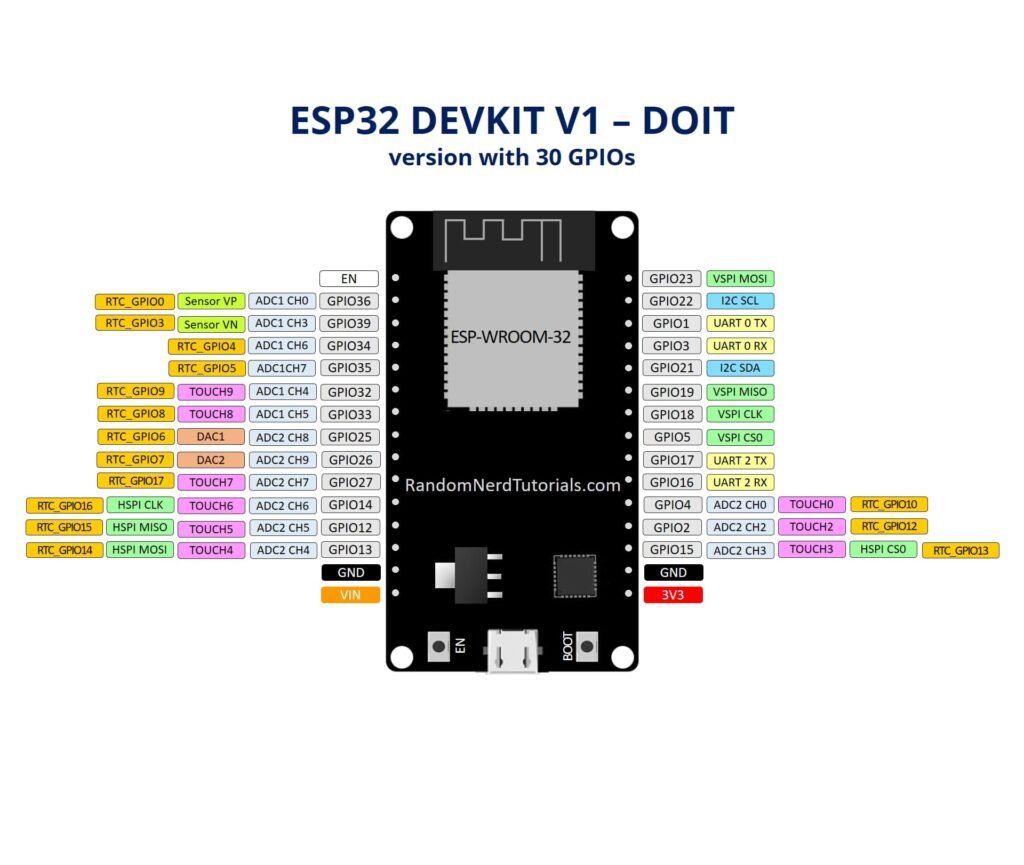
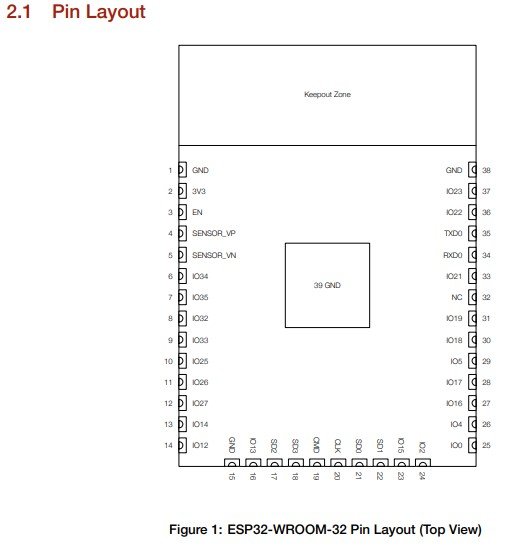
As this system includes the inbuild features like Bluetooth ,WIFI connectivity and GPIO pins it is reduce the extra components and cost of the system.
Features of ESP32:
- CPU: Xtensa dual-core (or single-core) 32-bit LX6 microprocessor, operating at 160 or 240 MHz and performing at up to 600 DMIPS.
- Ultra low power (ULP) co-processor.
- Memory: 520 KiB SRAM.
- Wi-Fi: 802.11 b/g/n
- Bluetooth: v4.2 BR/EDR and BLE (shares the radio with Wi-Fi)
- 12-bit SAR ADC up to 18 channels
- 2 × 8-bit DACs
- 10 × touch sensors (capacitive sensing GPIOs)
- 4 × SPI
- 2 × I²S interfaces
- 2 × I²C interfaces
- 3 × UART
As Snehal has developed a PCB for this controller I am going to used the same board for system.
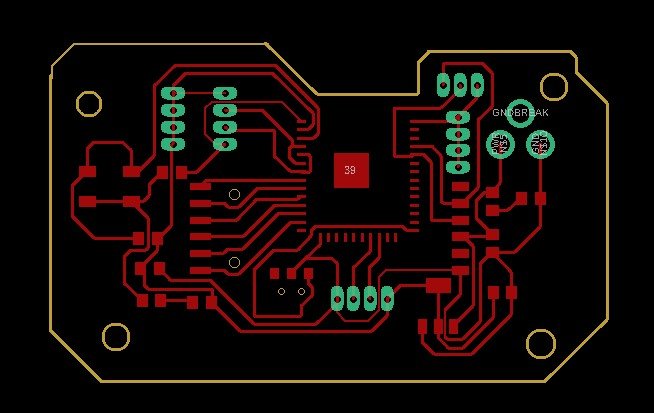
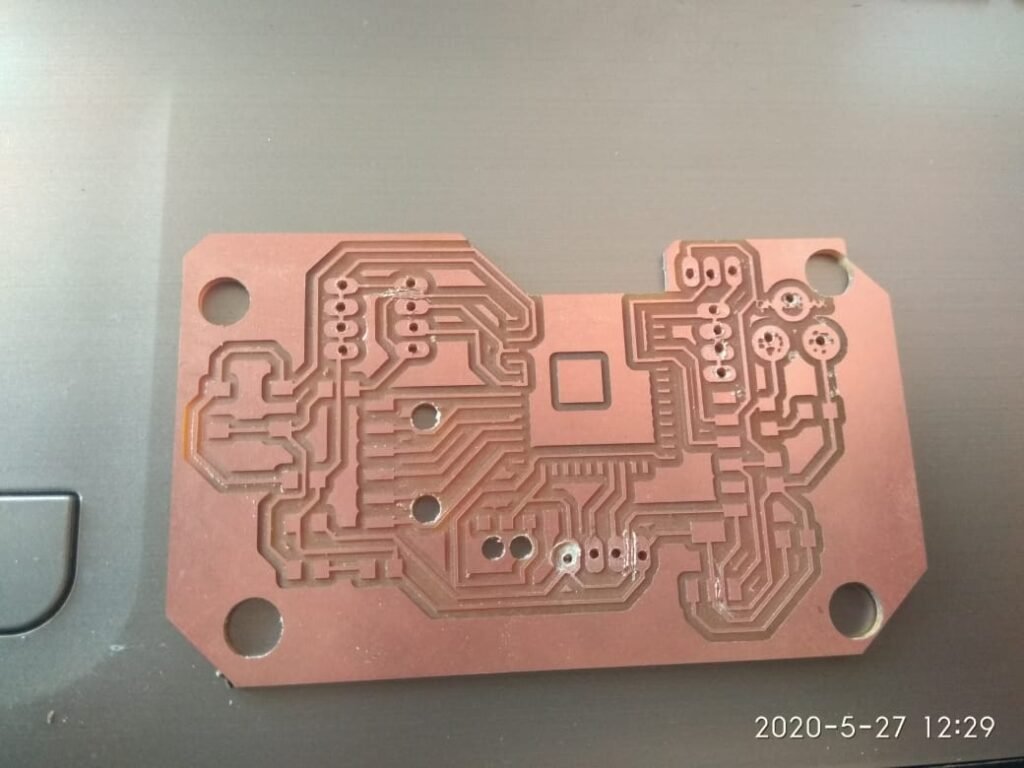
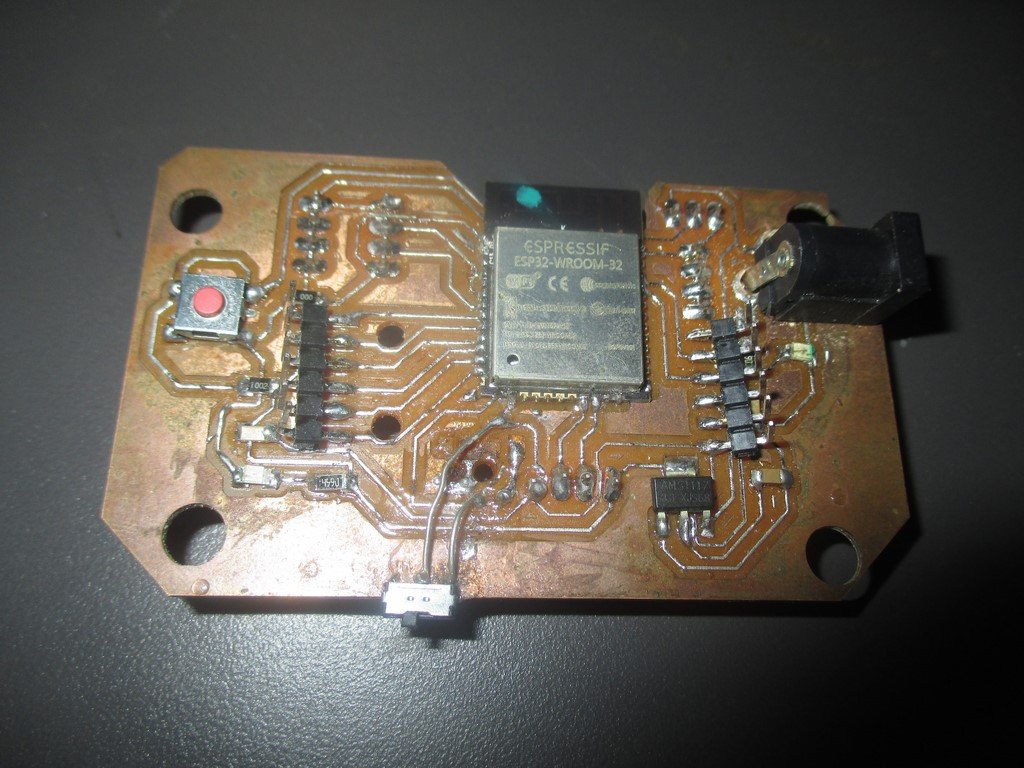
For designing of PCB and design files of eagle you can go through her website
Mobile app :
As system is IOT based the system should be smart and to monitor the water level ,we have included mobile app .Snehal developed the app but its very basic ,So I am going to change its user interface.
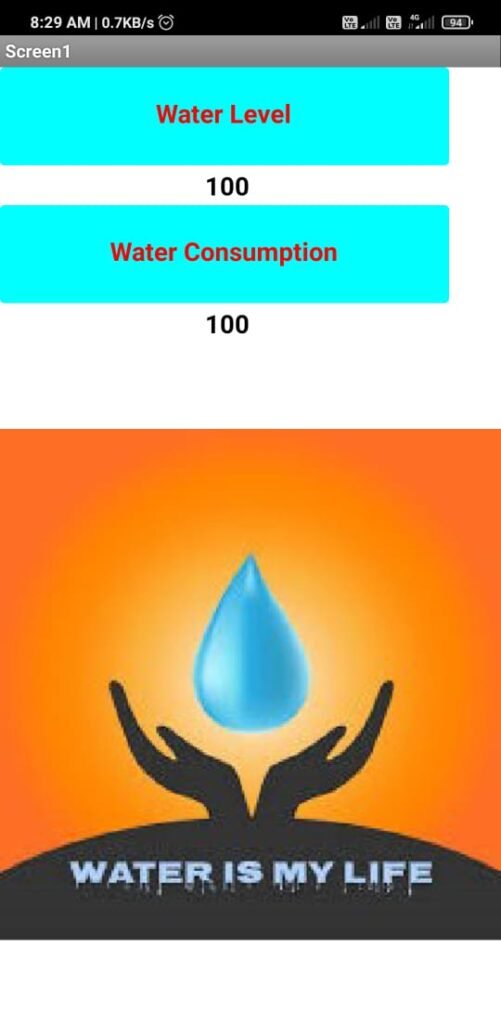
Downloadable apk file of mobile app
Programming:
Programming is key element of each electronic system,and as I am using ESP 32 board for the first time it seems very time consuming for me.
For programming I am using Arduino Ide ,First I have installed this library of Esp-32,then programmed the board .
Code:
#include <WiFi.h>
#include <WiFiClient.h>
#include <WiFiServer.h>
#include<dummy.h>
#define SONAR_NUM 1
#define PI 3.1415926535897932384626433832795
#include <Newping.h>
// Set web server port number to 80
WiFiServer server(80);
//String header;
//———– Enter you Wi-Fi Details———//
char ssid[] = “Workshop”; //SSID
char pass[] = “VIGYAN0030”; // Password
//——————————————-//
// ————— Tank details ————–//
//const int MAX_DISTANCE = 100; // Tank height in CM
//const int Depth1 = 25;// Water hold height in CM
const int MAX_DISTANCE = 122; //t //max distance to measure
const int Diameter1 = 110; //r //internal Diameter of tank 1 in cm
const int Depth1 = 100; //y //total depth of tank 1 in cm , from sensor to base inside
int Distance1;
int Litres1,WaterDepth1,WaterDepth2;
//——————————————-//
//—– minutes —–//
int minute = 2; // Data update in min.
//——————//
WiFiClient client;
//———– Channel Details ————-//
unsigned long Channel_ID = 1078169; // Channel ID
const int Field_number = 1; // To which field to write data (don’t change)
const int Field_number1 = 2; //
const char * WriteAPIKey = “Z4DKIZNQJ5LMQ28S”; // Your write API Key
const char * ReadAPIKey= “ZGCV0KK8K8LID0SL”;
// —————————————-//
const int trigger = 32;
const int echo = 33;
long Time;
int y;
int i;
int x;
int distanceCM;
int resultCM;
//int tnk_lvl = 0;
//int sensr_to_wtr = 0;
const int Area1 = PI * ((Diameter1 / 2) * (Diameter1 / 2)); //area of base of tank 1
// Global variables
NewPing sonar[SONAR_NUM] = { // Sensor object array.
NewPing(trigger, echo, Depth1) // Each sensor’s trigger pin, echo pin, and max distance to ping.
};
void setup()
{
Serial.begin(115200);
pinMode(trigger, OUTPUT);
pinMode(echo, INPUT);
WiFi.mode(WIFI_STA);
ThingSpeak.begin(client);
}
void loop()
{
internet();
delay(1000);
measure();
Serial.print(“Distance1:”);
Serial.print(Distance1);
Serial.println(“cm”);
Serial.print(“WaterDepth2:”);
Serial.print(WaterDepth2);
Serial.println(“%”);
Serial.print(“Tank Level:”);
Serial.print(“WaterDepth1:”);
Serial.print(WaterDepth1);
Serial.print(“Volume:”);
Serial.print(Litres1);
upload();
}
void upload()
{
internet();
ThingSpeak.writeField(Channel_ID, Field_number,WaterDepth1,WriteAPIKey);
ThingSpeak.writeField(Channel_ID, Field_number1,Litres1,WriteAPIKey);
}
void measure()
{
delay(100);
digitalWrite(trigger, HIGH);
delayMicroseconds(10);
digitalWrite(trigger, LOW);
//Time = pulseIn(echo, HIGH);
//distanceCM = Time * 0.034;
//resultCM = distanceCM / 2;
Distance1 = sonar[0].ping_cm(); //get distance to the top of the water tank 1
//if (Distance1 >= Depth1 || Distance1 == 0 ) Distance1 = Depth1; //check it does not go negative
WaterDepth1= Depth1-Distance1;
WaterDepth2 = (WaterDepth1*100)/Depth1 ;
Litres1 = (Area1 * WaterDepth1)/1000;
//tnk_lvl = map(resultCM, sensr_to_wtr, MAX_DISTANCE, 100, 0);
//if (tnk_lvl > 100) tnk_lvl = 100;
//if (tnk_lvl < 0) tnk_lvl = 0;
}
void internet()
{
if (WiFi.status() != WL_CONNECTED)
{
Serial.print(“Attempting to connect to SSID: “);
Serial.println(ssid);
while (WiFi.status() != WL_CONNECTED)
{
WiFi.begin(ssid, pass);
Serial.print(“.”);
delay(5000);
}
Serial.println(“\nConnected.”);
}
}
Testing:
One trial at lab is done ,results be like
Results case 1: When water level is greater than the 8 Cm of tank then motor will be automatically off.
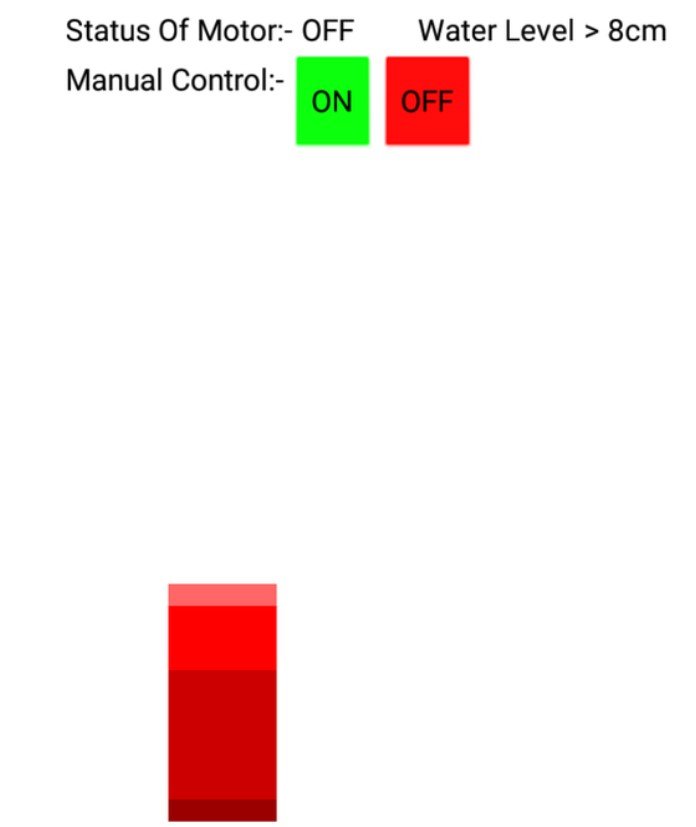
Results case 2: When water level is b than below the 8 Cm of tank then motor will be automatically on.
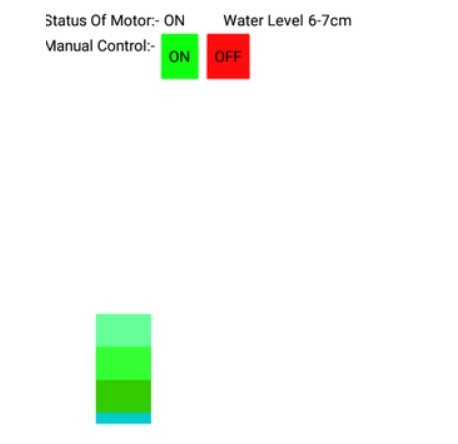